How to use Adonis, Postgresql, and Redis with Docker
Docker has become the standard for both development and production environments. As I've been working more and more with node and on my machine, I don't use anything but docker, I decided to create this little tutorial to teach you how to configure Adonis JS V5, Postgres, and Redis with Docker.
The first thing you need to have is the Docker installed on your computer, if you don't have it click here and follow the tutorial.
Within the root of your Adonis project create your Dockerfile.
FROM node:lts-alpine
RUN mkdir -p /home/node/app/node_modules
WORKDIR /home/node/app
COPY package.json yarn.* ./
RUN apk add --no-cache git
COPY . /home/node/app/
RUN chown -R node:node /home/node
RUN yarn
USER node
EXPOSE 3334
ENTRYPOINT ["node","ace","serve","--watch"]
Next, we'll create your docker-compose.yml also at the root of your adonis project.
version: '3'
services:
app:
build:
context: .
dockerfile: Dockerfile
volumes:
- .:/home/node/app
- /home/node/app/node_modules
ports:
- 3334:3334
networks:
- adonis
depends_on:
- pgsql
- redis
pgsql:
image: postgres:13
ports:
- '${PG_PORT:-5432}:5432'
environment:
PGPASSWORD: '${PG_PASSWORD:-secret}'
POSTGRES_DB: '${PG_DB_NAME}'
POSTGRES_USER: '${PG_USER}'
POSTGRES_PASSWORD: '${PG_PASSWORD:-secret}'
volumes:
- 'adonispgsql:/var/lib/postgresql/data'
networks:
- adonis
healthcheck:
test: ['CMD', 'pg_isready', '-q', '-d', '${PG_DB_NAME}', '-U', '${PG_USER}']
retries: 3
timeout: 5s
redis:
image: 'redis:alpine'
ports:
- '${REDIS_PORT:-6379}:6379'
volumes:
- 'adonisredis:/data'
networks:
- adonis
healthcheck:
test: ['CMD', 'redis-cli', 'ping']
mailhog:
image: 'mailhog/mailhog:latest'
ports:
- '${FORWARD_MAILHOG_PORT:-1025}:1025'
- '${FORWARD_MAILHOG_DASHBOARD_PORT:-8025}:8025'
networks:
- adonis
networks:
adonis:
driver: bridge
volumes:
adonispgsql:
driver: local
adonisredis:
driver: local
You will need to edit your .env file connections, as now your database and Redis will no longer connect to an IP but to the "docker internal network domain".
PORT=3000
HOST=0.0.0.0 // rede local
NODE_ENV=development
APP_KEY=LbJtLsxd5ElUo5KRVtpDzbs6BfB6t2pg
DRIVE_DISK=local
SMTP_HOST=localhost
SMTP_PORT=587
SMTP_USERNAME=
SMTP_PASSWORD=
REDIS_CONNECTION=local
REDIS_HOST=redis // o host passa a se chamar redis
REDIS_PORT=6379
REDIS_PASSWORD=
DB_CONNECTION=pg
PG_HOST=pgsql // o host passa a se chamar pgsql
PG_PORT=5432
PG_USER=adonis
PG_PASSWORD=adonis
PG_DB_NAME=adonis
Now you need to build your docker image and upload the container, for that first run the build command:
docker build .
After your image is built, let's upload the containers:
docker-compose up -d
With that, when you access http://localhost:3000 you should access your adonis project from the docker and no longer from your local machine.
Important To perform migrations and seed, you must access the app's container and execute the commands from within the container. Outwardly it won't work.
Extra If you want a tool to monitor your containers, I recommend using ctop.
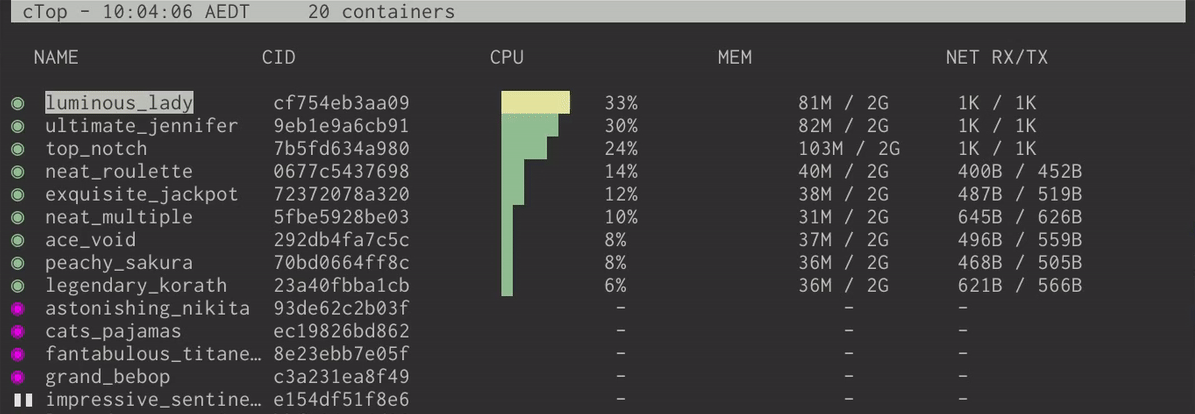